
Overview
Imagine a service that acts as a revolving door for all your API functionalities in AWS. That is how Amazon API Gateway fundamentally operates. API Gateway is a versatile utility that enables developers to create, publish and monitor APIs that handle workloads between clients and AWS services at scale. In this step-by-step tutorial, you will learn how to create a REST Endpoint using API Gateway, with Lambda serving requests at the backend. We start by creating a Lambda function using Python as the basic handler. From there, we create an API Gateway resource that acts as a public-facing REST Endpoint, which will be attached to the Lambda function. Finally, we will test the configuration by invoking the REST endpoint through the browser and verifying that the Lambda function serves the content back.
Prerequisites
This serverless tutorial assumes that you are familiar with the AWS Console and have the necessary IAM permissions set up.
If you are not signed up with an AWS account, first click on Activate Your AWS Account and then Create Your First IAM Admin User to follow detailed instructions on how to get started.
Step-by-step guide
Create a Lambda function
To create the Lambda function from scratch:
Open the Lambda console at https://console.aws.amazon.com/lambda/.
Do one of the following:
If the welcome page appears, choose Get Started Now and then choose Create function.
If the Lambda > Functions list page appears, choose Create function.
Choose Author from scratch.
In the Author from scratch pane, do the following:
For Function Name, enter TransactionProcessor as the Lambda function name.
For Runtime, choose Python 3.6.
For Execution role under Permissions, choose Create a new role with basic Lambda permissions.
Choose Create function.
Code the Lambda function with Python
The Python code consists of four steps undertaken by the Lambda function:

Copy the code below the following image and paste it in the lambda_function.py file in the Code source section under the Code tab of the Lambda > Functions>TransactionProcessor page.
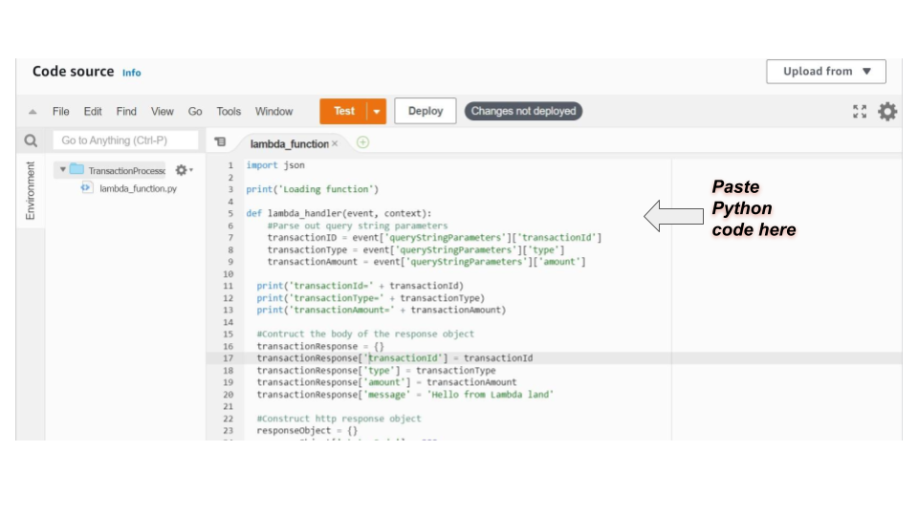
import json print('Loading function') def lambda_handler(event, context): #Parse out query string parameters transactionID = event['queryStringParameters']['transactionId'] transactionType = event['queryStringParameters']['type'] transactionAmount = event['queryStringParameters']['amount'] print('transactionId=' + transactionId) print('transactionType=' + transactionType) print('transactionAmount=' + transactionAmount) #Contruct the body of the response object transactionResponse = {} transactionResponse['transactionId'] = transactionId transactionResponse['type'] = transactionType transactionResponse['amount'] = transactionAmount transactionResponse['message' = 'Hello from Lambda land' #Construct http response object responseObject = {} responseObject['statusCode'] = 200 responseObject['headers'] {} responseObject['headers']['Content-Type'] = application/json responseOnject['body'] = json.dumps(transactionResponse) #Return the response object return responseObject
Click Save when done.
Integrate API Gateway with Lambda
Now that the Lambda function has been created, it is ready to be integrated with the API Gateway to activate its API functionalities. The API calls the Lambda function using an HTTP method.
To create an API with our custom Lambda function:
Sign in to the API Gateway console at https://console.aws.amazon.com/apigateway
If this is your first time using API Gateway, you see a page that introduces you to the features of the service. Under REST API, choose Build. When the Create Example API popup appears, choose OK.
If this is not your first time using API Gateway, choose Create API. Under REST API, choose Build. a. Choose New API. b. Enter a name in API Name. c. Optionally, add a brief description in Description. d. Choose Create API.
Choose the root resource (/) under Resources. From the Actions menu, choose Create Resource. a. Type transactions for Resource Name. b. Leave Resource Path as default (should be transactions.
c. Choose Create Resource.
With the newly created /{transactions} resource highlighted, choose Create Method from Actions. a. Choose GET from the HTTP method drop-down menu. b. To save the setting, choose the check mark.
In Method Execution, for the /{transactions} GET method, do the following:
Choose Lambda Function for Integration type.
Check the Use Lambda Proxy integration box.
Type the name of the Lambda function in Lambda Function. In our case, you will be prompted to choose the name we had given earlier for our Lambda function - TransactionProcessor.
Leave the Use Default timeout box checked.
Choose Save.
Choose OK in the Add Permission to Lambda Function popup to have API Gateway set up the required access permissions for the API to invoke the integrated Lambda function.
Deploy the API
We have set up the API, now it is time to test it. To do that, we need to create a stage where the API will be deployed. The stage logically separates your environment and creates a snapshot of the current API. You should note that if any change made to the API, you must redeploy it either to its existing stage or a new stage to effect the changes made.
To deploy the API to a stage:
Choose the API from the APIs pane or choose a resource or method from the Resources pane. Choose Deploy API from the Actions drop-down menu.
For Deployment stage, choose New Stage.
- For Stage name, type a name; for example, test.
Choose Deploy.
After the API is successfully deployed, you see the API's base URL (the default host name plus the stage name) displayed as Invoke URL at the top of the Stage Editor. The general pattern of this base URL is https://`api-id`.`region`.amazonaws.com/`stageName` . For example, the base URL of the API (beags1mnid) created in the us-west-2 region and deployed to the test stage is https://beags1mnid.execute-api.us-west-2.amazonaws.com/test.
Test the API
There are several ways you can test a deployed API. For GET requests using only URL path variables or query string parameters, you can type the API resource URL in a browser.
Test the API using in a web browser:
Copy the Invoke URL and paste it in your web browser
Append the end of the URL with "/transactions?transactionId=5&+type=PURCHASE&amount=500"
- Press Enter
If everything is configured correctly, you will see a JSON response that has these same values mirrored like the image below.
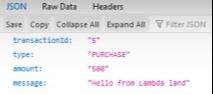
Clean-up
If you do not need the resources you have created, it is best practice to delete them to avoid incurring unnecessary charges to your account.